This is how a Javascript engine works
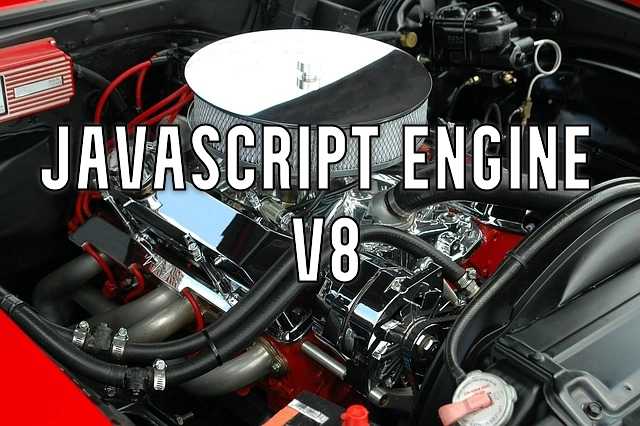
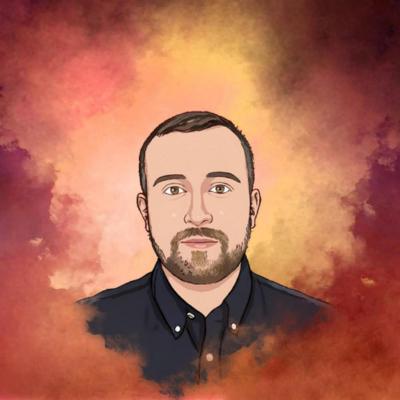
Intro
A JavaScript engine is a program that converts code into machine-readable instructions which can be executed by the computer's hardware. Modern JavaScript engines no longer just interpret code, they compile it. V8 is a JavaScript engine developed by Google for Chrome and Chromium web browsers. V8 is written in C++, it is portable and runs on Mac, Windows, Linux and other systems. Because of it's popularity, V8 will be the subject to disect in this article.
What does a running JavaScript engine do?
Works in cycles where a cycle has 3 steps. Parsing, compilation and execution.
Parsing the code
During the parsing steps, the code is tokenized, meaning that the broken down into small separate units which are understood by the engine. The syntax parser converts the code into an Abstract Syntax Tree (AST). Take this example:
const myName = 'George';
The Abstract Syntax Tree for the line of code above will look like this.
{
"type": "Program",
"start": 0,
"end": 24,
"body": [
{
"type": "VariableDeclaration",
"start": 0,
"end": 24,
"declarations": [
{
"type": "VariableDeclarator",
"start": 6,
"end": 23,
"id": {
"type": "Identifier",
"start": 6,
"end": 12,
"name": "myName"
},
"init": {
"type": "Literal",
"start": 15,
"end": 23,
"value": "George",
"raw": "'George'"
}
}
],
"kind": "const"
}
],
"sourceType": "module"
}
The parsing example above is generated using acorn. A JavaScript parser.
A range of errors can be caught at this step.
Let's say that we forget to provide a variable name.
const = 'george';
You will see in the console an error like this.
Uncaught SyntaxError: Unexpected token '='
Once the whole program has been parsed succesfully, without errors, the next step is to compile the code.
Compiling the code
This is the part where the engine converts human-readable code to machine code. JavaScript V8 engine uses a compiler and an interpreter and follows Just in Time(JIT) compilation algorithms. The engine finds patterns such as frequently executed functions, frequently used variables, and compiles them to improve performance. If the performance is affected or if the parameters passed to the function change their type, then the V8 simply decompiles the compiled code and falls back to the interpreter.
Executing the code
In the third step, the byte code is executed by using the Memory heap and the Call Stack of the V8 engine’s runtime environment. The memory Heap is the place where all the variables, objects and functions (callable objects) are assigned memory. The Call Stack is the place where each individual functions, when invoked, is pushed to the stack before execution. The V8 engine is created with performance and optimization in mind and it is trying continously to free up the memory heap, by clearing out unused functions and unreferenced objects using a process known as automatic garbage collection.
We have an article which goes deeper into explaining the garbage collection, so please check it out, Garbage collection.
This is a high overview of how a JavaScript engine works. Thank you.