Garbage Collection in JavaScript
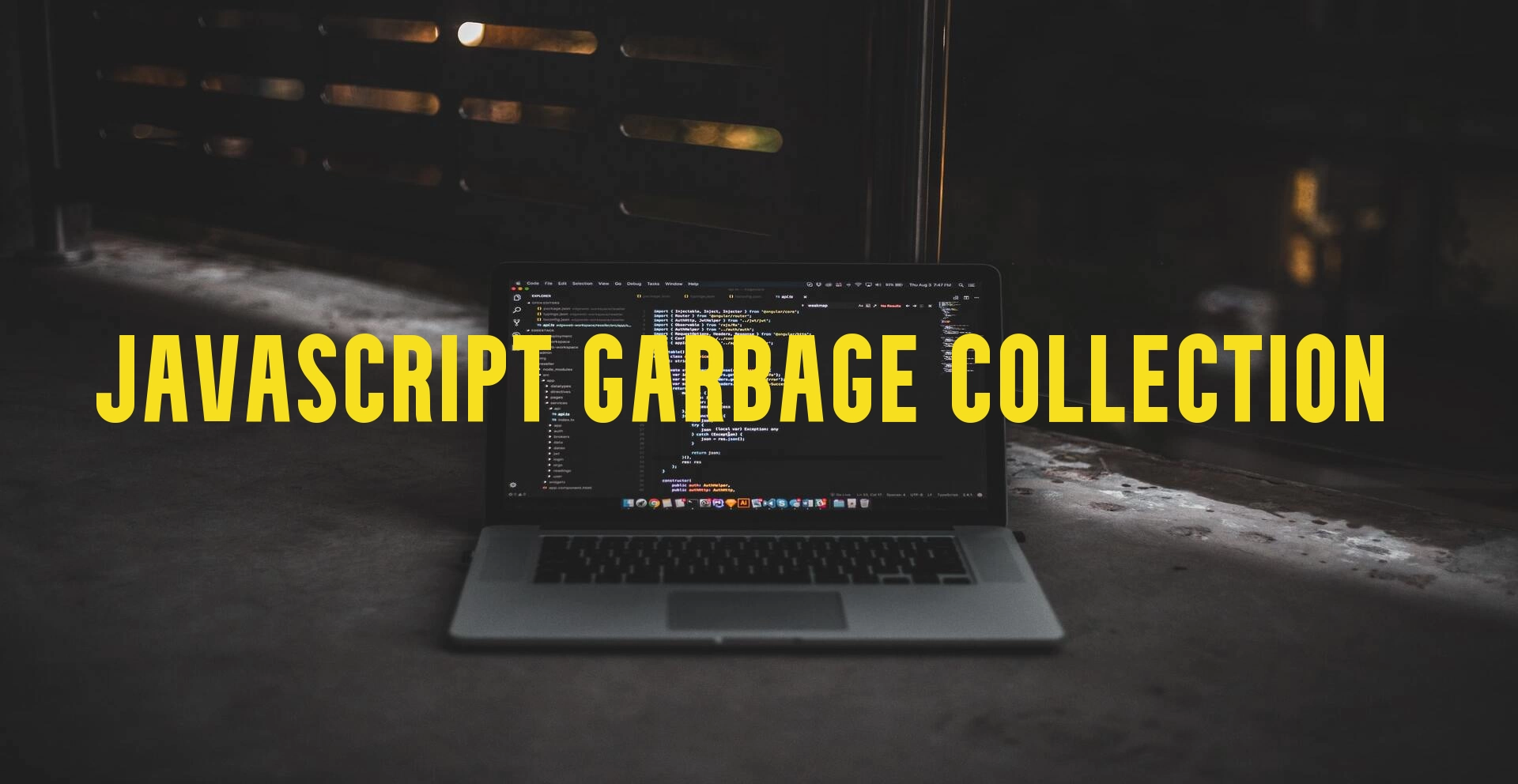
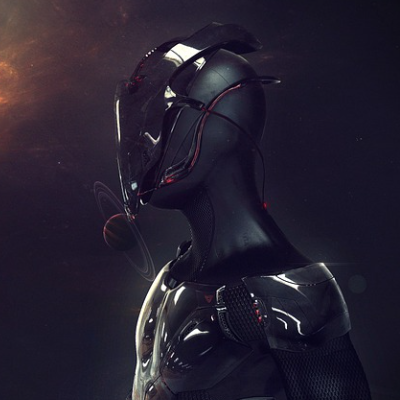
The data created in JavaScript needs memory in order to persist while the program is running. Have you ever asked yourself what happens with the data that is not needed anymore?
Not a sin if you have not. The engine does the job behind the scenes using a form of automatic memory management named garbage collector which has the task of discarding the data which is no more needed by the program.
The background process (GC) is monitoring all the objects and frees the space occupied by those objects that have become unreachable.
Here is an example.
A literal object and a variable pointing to it.
let motorcycle = {
makeAndModel: 'Honda CBR f4i',
};
At this point, the object occupies a place in computer memory.
If we reassign the variable "motorcycle", the reference gets lost.
motorcycle = undefined;
At this point the object is not reachable anymore, there is no pointer towards that object so the program has no use to it.
Can you guess what is happening with our object in this case?
You are right, it's getting garbage collected.
The basic garbage collection algorithm is called “mark-and-sweep” and performs the following steps:
1. The garbage collector takes the roots(inherently reachable values) and marks them to recall them later.
2. As a second step, the process visits and marks all references from roots.
3. Then goes over the marked objects and marks their references to be able to prevent visiting the same objects again, for performance reason.
4. Step 3 is happening recursively until all the objects are visited.
5. As a final step, all unreachable objects get garbage collected.
This is how garbage collection works. The JavaScript engines apply many other optimizations on top of this process to make it run faster.
Thank you if you've managed to follow so far.