TypeScript Exception - Property does not exist on type window
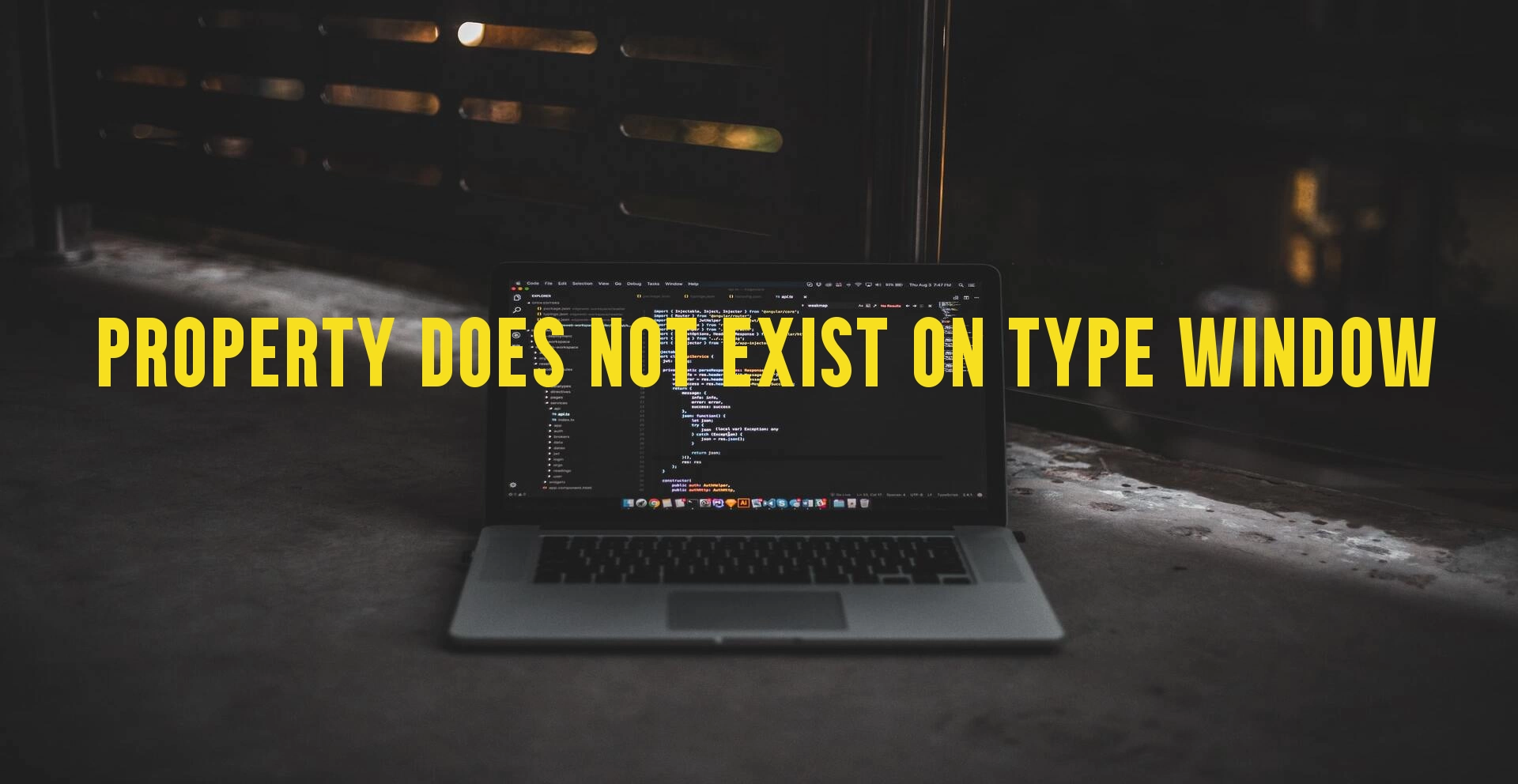
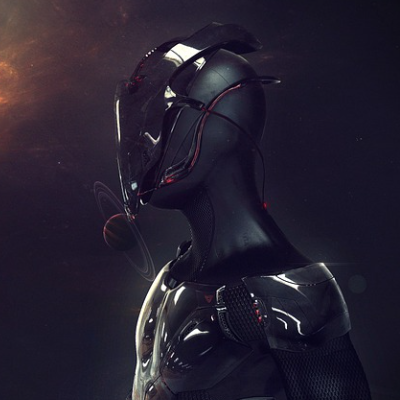
Fix TypeScript Exception "Property does not exist on type 'Window & typeof globalThis'
This error appears when we access a property that does not exist on the Window interface. To solve the error, extend or create the Window interface in a .d.ts file by adding the property you intend to access or to create on the window object.
Let's say that you attempt to create a new global property like this:
const test = window.testingAround;
The TypeScript compiler will immediately catch this probme and will report the message: Property 'testingAround' does not exist on type 'Window & typeof globalThis'.
In your project's root directory create a directory named "types" and inside of it create a new file named "index.d.ts".
Inside the file add this code
export { };
declare global {
interface Window {
testingAround: object; // In this case we need testingAround property, add the property you need instead
}
}
TypeScript will merge this declaration with the original Window interface. Next time when you use the window object you will be able to access properties from both declarations.
For the case when you don't know the type of the property you may use "any".
export { };
declare global {
interface Window {
testingAround: any; // Typed as any
}
}
If you still have an issue it may be the case that you need to amend "tsconfig.json" file. In the compilerOptions object, set the "typeRoots" to point the the place where you've created the type definition.
{
"compilerOptions": {
// ... other rules
"typeRoots": ["./node_modules/@types", "./types"]
}
}
If you are asking yourself what is the purpose of export {}
This is meant to let the compiler know that we have this file as an external module. We are required to do this is order to be able to augment the global scope.
I hope this helped you to get over the error and to continue working on your amazing App.