Implicit and explicit coercion in JavaScript
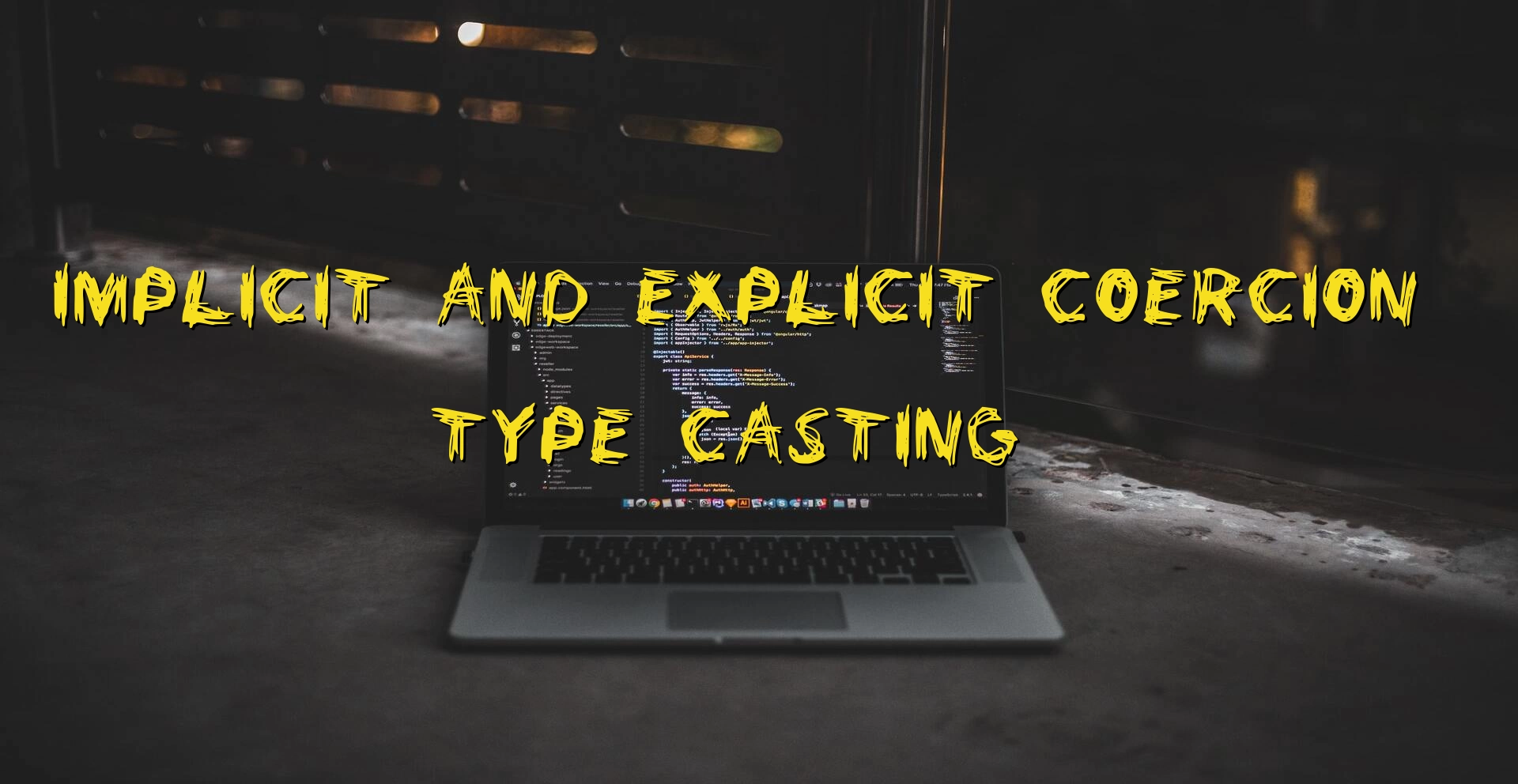
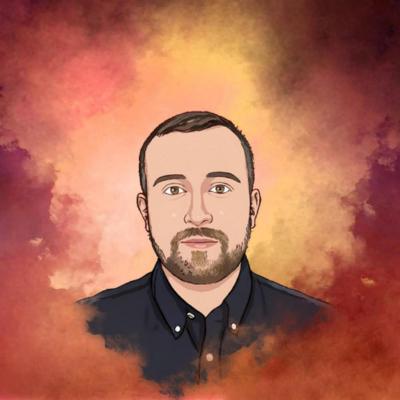
Three types of conversion
JavaScript is a weakly-typed language, values can also be converted between different types automatically. This is what we call implicit type coercion. We also have the ability to convert values in a more implicit way using the "boxing" technique.
First we need to understand that there are exactly three types of conversions possible:
- - to string
- - to boolean
- - to number
The second part, the conversion logic for primitives and objects is different, both primitives and objects can only be converted in those three types mentioned above but the case for objects is more complex involving methods like "toString()" and "valueOf()". Because coercion rules for objects becomes quicly a very long topic, this case is not covered in this article.
I. String conversion
To explicitly convert values to a string apply the "boxing" technique using the String() class. Implicit coercion is triggered by the binary + operator, when any operand is a string.
String(2609) // explicit => result: "2609"
2609 + '' // implicit => result: "2609"
With explicit coercion all primitive values are getting converted to string.
// RESULTS:
String(2609) // '2609'
String(-152.3) // '-152.3'
String(null) // 'null'
String(undefined) // 'undefined'
String(true) // 'true'
String(false) // 'false'
String(Symbol('a new symbol')) // 'Symbol(a new symbol)'
II. Boolean conversion
To explicitly convert to a boolean use the Boolean() function. Implicit conversion happens in logical context, or is triggered by logical operators ( || && !).
Boolean(2) // explicit => result: true
if (2) { ... } // implicit due to logical context
!!1 // implicit due to logical operator => result: true
In the next two examples the values are coerced internally to boolean but the result is the original type, the one before evaluation.
2 || 'hi George' // implicit due to logical operator => result: 2
2 && 'hi George' // implicit due to logical operator => result: "hi George"
All the falsey values ... guess what? evaluate to false, conversly the truthy values to true.
Boolean('') // false
Boolean(0) // false
Boolean(-0) // false
Boolean(NaN) // false
Boolean(null) // false
Boolean(undefined) // false
Boolean(false) // false
III. Conversion to Numbers
Number() function is used for explicit coercion. When converting a string to a number, the engine returns NaN if the trimmed string does not represent a valid number. If string is empty, it returns 0. When dealing with null and undefined, null becomes 0, whereas undefined becomes NaN.
Number('2609') // explicit => result: 2609
+'2609' // implicit => result: 2609
123 != '2509' // implicit=> '2509' becomes 2509
4 > '5' // implicit
5/null // implicit
true | 0 // implicit
Other examples of explicit coercions with results.
// RESULTS
Number(null) // 0
Number(undefined) // NaN
Number(true) // 1
Number(false) // 0
Number(" 12 ") // 12
Number("-12.34") // -12.34
Number("\n") // 0
Number(" 12s ") // NaN
Number(123) // 123
Helpful links: