Arrow function limitations in JavaScript
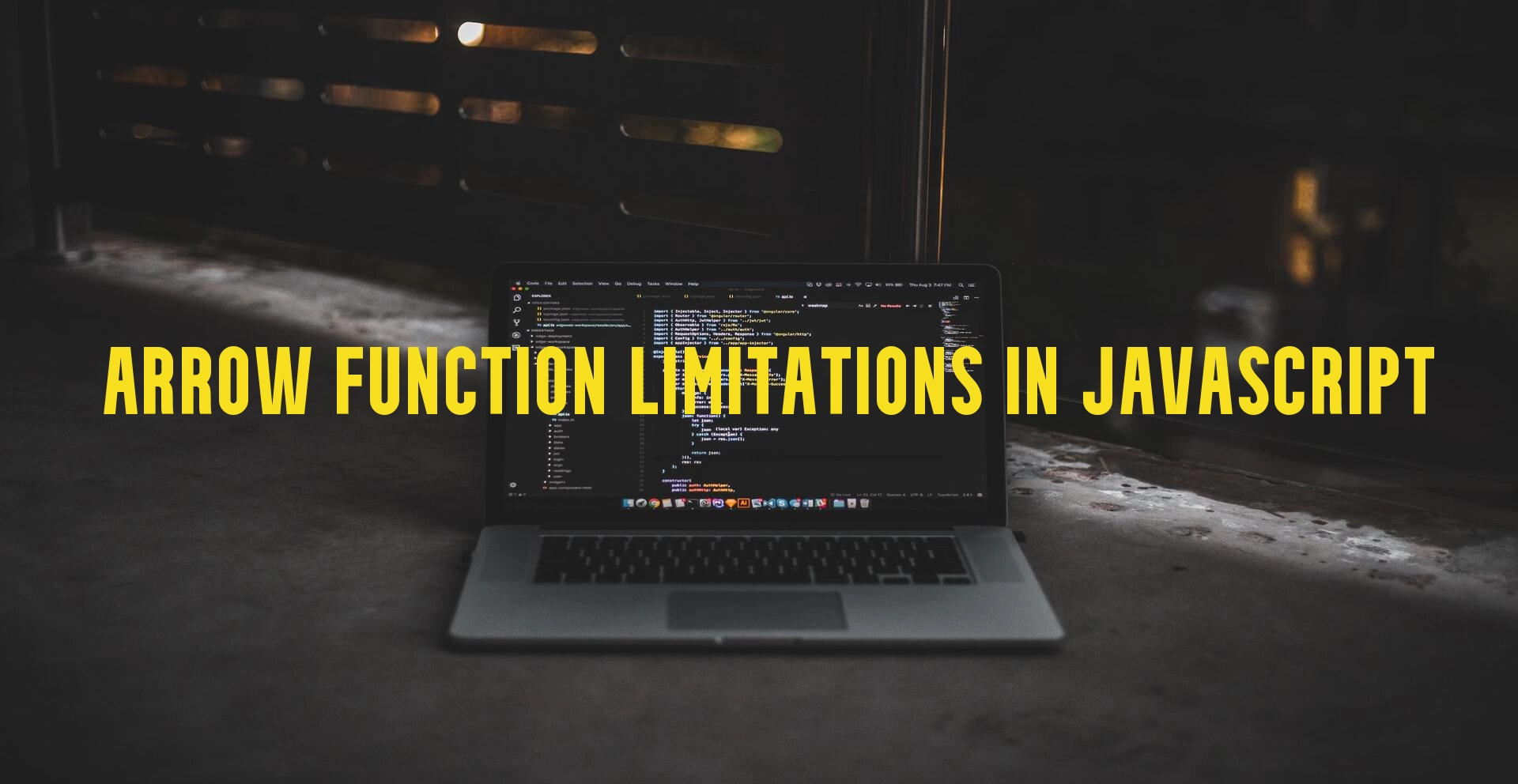
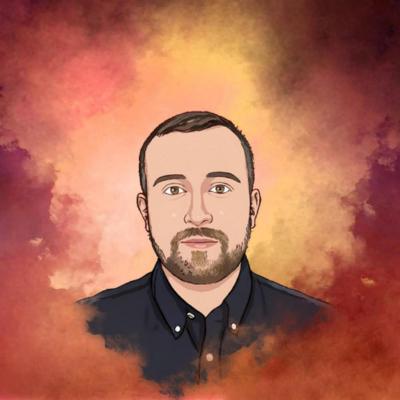
An arrow function should not be used as method.
Arrow function expressions are best suited for non-method functions. For example, after we instatiate the test object, the increment method does not have its own "this" so it will borrow the Window object in this case.
See the example below.
function DinoCount() {
this.count = 0;
}
DinoCount.prototype.increment = () => {
console.log(this, this.count);
return this.count += 1;
};
DinoCount.prototype.current = () => {
return this.count;
}
let test = new DinoCount();
test.increment();
// Output
VM904:6 Window {0: global, 1: global, 2: global, window: Window, self: Window, document: document, name: '', location: Location, …} NaN
There is an exception though, if we had a static field that would work. The class has its own "this" so the "this" from within the arrow function will default as expected to the enclosing scope "this".
function DinoCount() {
exempluStatic = 'As expected';
this.count = 0;
}
DinoCount.prototype.increment = () => {
console.log(this.exempluStatic);
return this.count += 1;
};
DinoCount.prototype.current = () => {
return this.count;
}
let test = new DinoCount();
test.increment();
// Output
VM227:9 As expected
An arrow function cannot be used as constructors.
Trying to instantiate a class with he "new" keyword will throw an error. Arrow functions cannot be used as constructors.
const Dino = () => { };
const dinoTest = new Dino();
// Output
VM75:2 Uncaught TypeError: Dino is not a constructor
at <anonymous>:2:14
An arrow function can not use yield within its body.
The yield keyword cannot be used in an arrow function's body (except when permitted within functions further nested within it). As a consequence, arrow functions cannot be used as generators.
Arrow function cannot be suitable for call, apply and bind methods.
"call", "apply" and "bind" methods are not suitable the invoked on arrow functions. These were designed to allow methods to execute within different scopes. Arrow functions establish the "this" keyword based on the scope the arrow function is defined within.
const dinoTest = {
num: 100,
};
// Arrow Function
const addNr = (a, b, c) => this.num + a + b + c;
// call
console.log(addNr.call(dinoTest, 1, 2, 3));
// apply
const arr = [1, 2, 3];
console.log(addNr.apply(dinoTest, arr));
// bind
const bound = addNr.bind(dinoTest);
console.log(bound(1, 2, 3));
// Output
VM133:15 NaN
VM133:19 NaN
VM133:23 NaN